Undirected Grid GraphΒΆ
2D and 3D undirected with simple neighborhood (4-neighborhood in 2D, 6-neighborhood in 3D)
from __future__ import print_function
import nifty.graph
import pylab
2D undirected grid graph
shape = [3, 3]
graph = nifty.graph.undirectedGridGraph(shape)
print("#nodes", graph.numberOfNodes)
print("#edges", graph.numberOfEdges)
print(graph)
Out:
#nodes 9
#edges 12
#Nodes 9 #Edges 12
iterate over nodes and the adjacency of each node
for node in graph.nodes():
print("u",node)
for v,e in graph.nodeAdjacency(node):
print(" v",v,"e",e)
Out:
u 0
v 1 e 0
v 3 e 6
u 1
v 0 e 0
v 2 e 1
v 4 e 7
u 2
v 1 e 1
v 5 e 8
u 3
v 0 e 6
v 4 e 2
v 6 e 9
u 4
v 1 e 7
v 3 e 2
v 5 e 3
v 7 e 10
u 5
v 2 e 8
v 4 e 3
v 8 e 11
u 6
v 3 e 9
v 7 e 4
u 7
v 4 e 10
v 6 e 4
v 8 e 5
u 8
v 5 e 11
v 7 e 5
iterate over edges and print the endpoints
for edge in graph.edges():
print("edge ",edge, "uv:", graph.uv(edge))
Out:
edge 0 uv: (0, 1)
edge 1 uv: (1, 2)
edge 2 uv: (3, 4)
edge 3 uv: (4, 5)
edge 4 uv: (6, 7)
edge 5 uv: (7, 8)
edge 6 uv: (0, 3)
edge 7 uv: (1, 4)
edge 8 uv: (2, 5)
edge 9 uv: (3, 6)
edge 10 uv: (4, 7)
edge 11 uv: (5, 8)
get the uv-ids /endpoints for all edges simultaneous as a numpy array
uvIds = graph.uvIds()
print(uvIds)
Out:
[[0 1]
[1 2]
[3 4]
[4 5]
[6 7]
[7 8]
[0 3]
[1 4]
[2 5]
[3 6]
[4 7]
[5 8]]
get the coordinates of a node
for node in graph.nodes():
print("node",node,"coordiante",graph.nodeToCoordinate(node))
Out:
node 0 coordiante [0, 0]
node 1 coordiante [0, 1]
node 2 coordiante [0, 2]
node 3 coordiante [1, 0]
node 4 coordiante [1, 1]
node 5 coordiante [1, 2]
node 6 coordiante [2, 0]
node 7 coordiante [2, 1]
node 8 coordiante [2, 2]
get the node of a coordinate
for x0 in range(shape[0]):
for x1 in range(shape[1]):
print("coordiante",[x0,x1],"node",graph.coordianteToNode([x0,x1]))
Out:
coordiante [0, 0] node 0
coordiante [0, 1] node 1
coordiante [0, 2] node 2
coordiante [1, 0] node 3
coordiante [1, 1] node 4
coordiante [1, 2] node 5
coordiante [2, 0] node 6
coordiante [2, 1] node 7
coordiante [2, 2] node 8
plot the graph: needs networkx
nifty.graph.drawGraph(graph)
pylab.show()
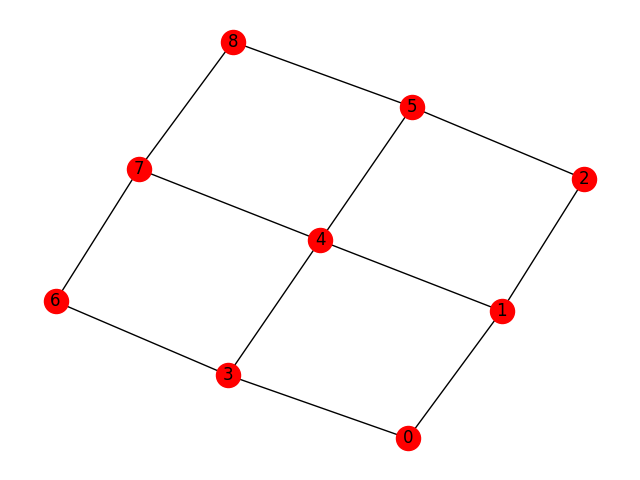
Total running time of the script: ( 0 minutes 0.047 seconds)