Undirected Graph: Simple ExampleΒΆ
Very simple example how to use undirected graphs
from __future__ import print_function
import nifty.graph
import numpy
import pylab
2D undirected grid graph
numberOfNodes = 5
graph = nifty.graph.undirectedGraph(numberOfNodes)
print("#nodes", graph.numberOfNodes)
print("#edges", graph.numberOfEdges)
print(graph)
Out:
#nodes 5
#edges 0
#Nodes 5 #Edges 0
insert edges
graph.insertEdge(0,1)
graph.insertEdge(0,2)
insert multiple edges at once
uvIds = numpy.array([[0,3],[1,2],[1,4],[1,3],[3,4]])
graph.insertEdges(uvIds)
iterate over nodes and the adjacency of each node
for node in graph.nodes():
print("u",node)
for v,e in graph.nodeAdjacency(node):
print(" v",v,"e",e)
Out:
u 0
v 1 e 0
v 2 e 1
v 3 e 2
u 1
v 0 e 0
v 2 e 3
v 3 e 5
v 4 e 4
u 2
v 0 e 1
v 1 e 3
u 3
v 0 e 2
v 1 e 5
v 4 e 6
u 4
v 1 e 4
v 3 e 6
iterate over edges and print the endpoints
for edge in graph.edges():
print("edge ",edge, "uv:", graph.uv(edge))
Out:
edge 0 uv: (0, 1)
edge 1 uv: (0, 2)
edge 2 uv: (0, 3)
edge 3 uv: (1, 2)
edge 4 uv: (1, 4)
edge 5 uv: (1, 3)
edge 6 uv: (3, 4)
get the uv-ids /endpoints for all edges simultaneous as a numpy array
uvIds = graph.uvIds()
print(uvIds)
Out:
[[0 1]
[0 2]
[0 3]
[1 2]
[1 4]
[1 3]
[3 4]]
plot the graph: needs networkx
nifty.graph.drawGraph(graph)
pylab.show()
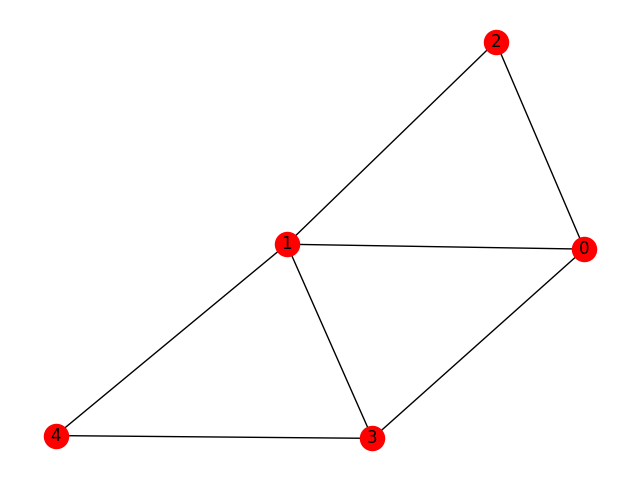
Total running time of the script: ( 0 minutes 0.192 seconds)